首页> 基础笔记 >Vue学习笔记 Vue学习笔记
vue动态组件保持存活(缓存)--keep-alive组件
作者:小萝卜 2024-03-20 【 Vue 】 浏览 183
简介当使用 <component :is="..."> 来在多个组件间作切换时,被切换掉的组件会被卸载。我们可以通过 <KeepAlive> 组件强制被切换掉的组件仍然保持“存活”的状态。
当使用 <component :is="..."> 来在多个组件间作切换时,被切换掉的组件会被卸载。我们可以通过 <KeepAlive> 组件强制被切换掉的组件仍然保持“存活”的状态。
<template>
<div class="container">
<!-- 使用属性绑定指令将指定的数据绑定到属性中 -->
<component v-bind:is="show"></component>
<button @click="transfer()">Transfer</button>
</div>
</template>
<script>
// 导入组件
import First from '@/components/First.vue';
import Last from '@/components/Last.vue';
export default {
// 定义数据
data() {
return {
show: 'First'
}
},
// 注册组件
components: {
First,
Last
},
// 定义事件处理函数
methods: {
transfer() {
if(this.show === 'First'){
this.show = 'Last';
}else{
this.show = 'First';
}
}
}
}
</script>
执行效果:
分析:在切换组件后,原组件将被销毁。再次切换为原组件时,由于组件经历了再生成的过程,组件中的数据都将处于初始状态。
keep-alive
在实现组件的动态切换功能时,你可能会想保持这些组件的状态,以避免反复重新渲染导致的性能问题。我们可以使用 keep-alive 元素达成该目的。
keep-alive 能够使被替换的组件不用经历组件的再生成过程,被替换的组件将处于缓存状态,等待用户的再次使用。keep-alive 的使用方式很简单,你仅需要使用 keep-alive 元素将需要被缓存的动态组件包裹起来。
<template>
<div class="container">
<keep-alive>
<!-- 使用属性绑定指令将指定的数据绑定到属性中 -->
<component v-bind:is="show"></component>
</keep-alive>
<button @click="transfer()">Transfer</button>
</div>
</template>
<script>
// 导入组件
import First from '@/components/First.vue';
import Last from '@/components/Last.vue';
export default {
// 定义数据
data() {
return {
show: 'First'
}
},
// 注册组件
components: {
First,
Last
},
// 定义事件处理函数
methods: {
transfer() {
if(this.show === 'First'){
this.show = 'Last';
}else{
this.show = 'First';
}
}
}
}
</script>
执行效果:
keep-alive属性include
keep-alive 元素拥有 include 属性,你可以通过向该属性提交组件名来指定需要被缓存的组件。如果有多个组件需要被缓存,请使用逗号分隔组件名。
<template>
<div class="container">
<!-- 指定 First 组件需要被缓存 -->
<keep-alive include="First">
<!-- 使用属性绑定指令将指定的数据绑定到属性中 -->
<component v-bind:is="show"></component>
</keep-alive>
<button @click="transfer()">Transfer</button>
</div>
</template>
执行效果:
keep-alive属性exclude
keep-alive 元素拥有 exclude 属性,你可以通过向该属性提交组件名来指定不需要被缓存的组件。如果有多个组件不需要被缓存,请使用逗号分隔组件名。
<template>
<div class="container">
<!-- 指定 First 组件不需要被缓存 -->
<keep-alive exclude="First">
<!-- 使用属性绑定指令将指定的数据绑定到属性中 -->
<component v-bind:is="show"></component>
</keep-alive>
<button @click="transfer()">Transfer</button>
</div>
</template>
<script>
// 导入组件
import First from '@/components/First.vue';
import Last from '@/components/Last.vue';
export default {
// 定义数据
data() {
return {
show: 'First'
}
},
// 注册组件
components: {
First,
Last
},
// 定义事件处理函数
methods: {
transfer() {
if(this.show === 'First'){
this.show = 'Last';
}else{
this.show = 'First';
}
}
}
}
</script>
执行效果:
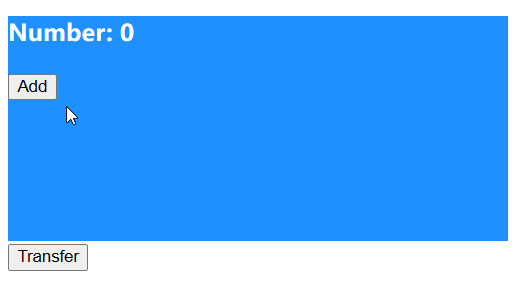
keep-alive组件生命周期钩子
在组件被缓存或被激活的时候将触发相应的生命周期钩子(生命周期函数)。
当组件被激活时将触发 activated 生命周期钩子。
当组件被缓存时将触发 deactivated 生命周期钩子。
父组件:
<template>
<div class="container">
<keep-alive>
<!-- 使用属性绑定指令将指定的数据绑定到属性中 -->
<component v-bind:is="show"></component>
</keep-alive>
<button @click="transfer()">Transfer</button>
</div>
</template>
<script>
// 导入组件
import First from '@/components/First.vue';
import Last from '@/components/Last.vue';
export default {
// 定义数据
data() {
return {
show: 'First'
}
},
// 注册组件
components: {
First,
Last
},
// 定义事件处理函数
methods: {
transfer() {
if(this.show === 'First'){
this.show = 'Last';
}else{
this.show = 'First';
}
}
}
}
</script>
子组件 First.vue
<template>
<div class="container">
<h3>Number: {{ num }}</h3>
<button @click="add()">Add</button>
</div>
</template>
<script>
export default {
// 定义数据
data() {
return {
num: 0
}
},
// 定义事件调用函数
methods: {
add() {
this.num += 1;
}
},
// activated 生命周期对应的生命周期钩子
activated() {
console.log('【Activated】')
},
// deactivated 生命周期对应的生命周期钩子
deactivated() {
console.log('【Deactivated】')
}
}
</script>
<style scoped>
.container{
width: 400px;
height: 180px;
background-color: dodgerblue;
}
h3{
color: #fff;
}
</style>
执行效果:
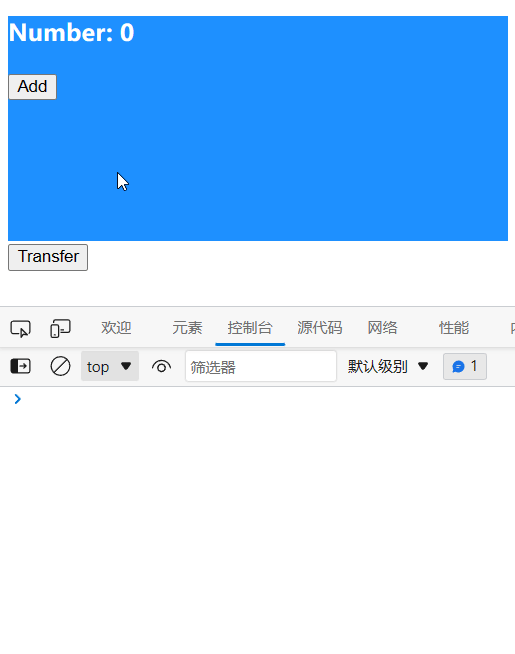
很赞哦! (0)
下一篇:vue动态组件基本介绍